Table Of Content

Structural design patterns provide different ways to create a Class structure (for example, using inheritance and composition to create a large Object from small Objects). The alchemist must be able to create both gold and copper coins and switching between them must be possible without modifying the existing source code. The factory pattern makes it possible by providing a static construction method which can be called with relevant parameters.
Structural Design Patterns in Java
If you still have some doubt on abstract factory design pattern in Java, please leave a comment. CarType will hold the types of car and will provide car types to all other classes. Defines the skeleton of an algorithm in the superclass but lets subclasses override specific steps of the algorithm without changing its structure. Mediator Method is a Behavioral Design Pattern, it promotes loose coupling between objects by centralizing their communication through a mediator object. Instead of objects directly communicating with each other, they communicate through the mediator, which encapsulates the interaction and coordination logic.
Use Cases of the Factory Method Design Pattern in Java
The pattern allows you to produce different types and representations of an object using the same construction code. From Lehman’s point of view, we can say that a factory is a place where products are created. In order words, we can say that it is a centralized place for creating products. Later, based on the order received, the appropriate product is delivered by the factory.
Concrete Creators
So far we learned what is Factory method design pattern and how to implement it. I believe now we have a fair understanding of the advantage of this design mechanism. Factory methods pervade toolkits and frameworks.The preceding document example is a typical use in MacApp and ET++. Adapter Method is a structural design pattern, it allows you to make two incompatible interfaces work together by creating a bridge between them. The Data Access Object (DAO) design pattern is used to decouple the data persistence logic to a separate layer.
Design Patterns
It is a creational design pattern that defines the process of the creation of an object. These patterns control the way we define and design the objects, as well as how we instantiate them. Factory pattern is most suitable where there is some complex object creation steps are involved. To ensure that these steps are centralized and not exposed to composing classes, factory pattern should be used.
Creational Design Patterns in Java
Now that we have super classes and sub-classes ready, we can write our factory class. Lets you define a family of algorithms, put each of them into a separate class, and make their objects interchangeable. Lets you produce families of related objects without specifying their concrete classes. A design pattern is a generic repeatable solution to a frequently occurring problem in software design that is used in software engineering.
The Solution — Factory Method:
This pattern treats both individual objects and compositions of objects it allow clients to work with complex structures of objects as if they were individual objects. The implementation of the bridge design pattern follows the notion of preferring composition over inheritance. Client code works with factories and products using abstract interfaces. It makes the same client code working with many product variants, depending on the type of factory object.
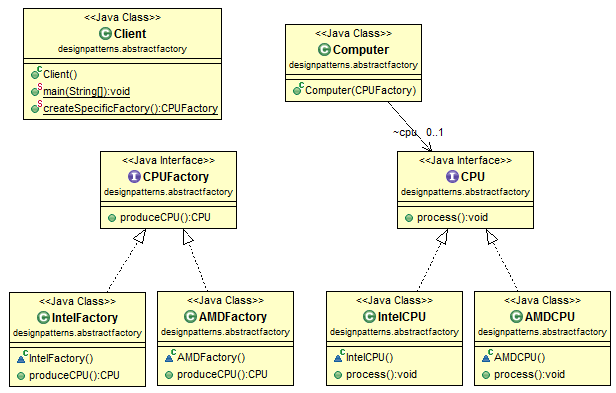
Having said that I want to invite you to partner me in clearing the space and providing the correct Design patterns as they are and not as they occur to you, me or any other author. I want to point out that the example you have put is neither of that. In the factory pattern , the factory class has an abstract method to create the product and lets the sub classes to create the concrete product.
[GSoC 2018] First Collector, Core object implementation – helloworlditsme - İTÜ
[GSoC 2018] First Collector, Core object implementation – helloworlditsme.
Posted: Mon, 11 Jun 2018 07:00:00 GMT [source]
Since every object consumes memory space that can be crucial for low memory devices, flyweight design pattern can be applied to reduce the load on memory by sharing objects. Bridge Method is a structural design pattern,it provide to design separate an object’s abstraction from its implementation so that the two can vary independently. First of all, it allows developers to support more objects with their factory class as times passes by, without affecting client code.
Then, through as many if statements required, we check which exact class should be used to serve the call. Sooner or later, a desktop program, mobile app, or some other type of software will inevitably become complex and start exhibiting certain kinds of problems. These problems are typically related to the complexity of our codebase, non-modularity, inability to separate certain parts from each other, etc.

It makes the system independent and how its objects are created, composed, and represented. Client code works with factories and products only through their abstract interfaces. This lets the client code work with any product variants, created by the factory object.
A factory (i.e. a class) will create and deliver products (i.e. objects) based on the incoming parameters. We know that we can have multiple catch blocks in a try-catch block code. Here every catch block is kind of a processor to process that particular exception. So when an exception occurs in the try block, it’s sent to the first catch block to process. If the catch block is not able to process it, it forwards the request to the next Object in the chain (i.e., the next catch block).
On all tutorials which I use, free and bought I never heard any of those teacher that they are mentioned any design pattern. I know they exist but how I see at the interview they ask about them. The prototype pattern is used when the Object creation is costly and requires a lot of time and resources, and you have a similar Object already existing. So this pattern provides a mechanism to copy the original Object to a new Object and then modify it according to our needs. The prototype design pattern mandates that the Object which you are copying should provide the copying feature. However, whether to use the shallow or deep copy of the object properties depends on the requirements and is a design decision.
For this reason, design patterns have become the de facto standard in the programming industry since their initial use a few decades ago due to their ability to solve many of these problems. In this article, we will dive deeper into one of these methodologies - namely, the Factory Method Pattern. This is an interface or an abstract class that declares the method(s) that need to be implemented by the concrete products. In the example provided, Currency is the product interface with the method getSymbol(). The solution is to reduce the code that constructs components across the framework into a single factory method and let anyone override this method in addition to extending the component itself.
This approach separates the object creation from the implementation, which promotes loose coupling and thus easier maintenance and upgrades. For example, to add a new product type to the app, you’ll only need to create a new creator subclass and override the factory method in it. As you can see, the factory is able to return any type of car instance it is requested for. It will help us in making any kind of changes in car making process without even touching the composing classes i.e. classes using CarFactory.
The client code calls the creation methods of a factory object instead of creating products directly with a constructor call (new operator). Since a factory corresponds to a single product variant, all its products will be compatible. This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object.
No comments:
Post a Comment